Navigating Shell for Productivity and Profit
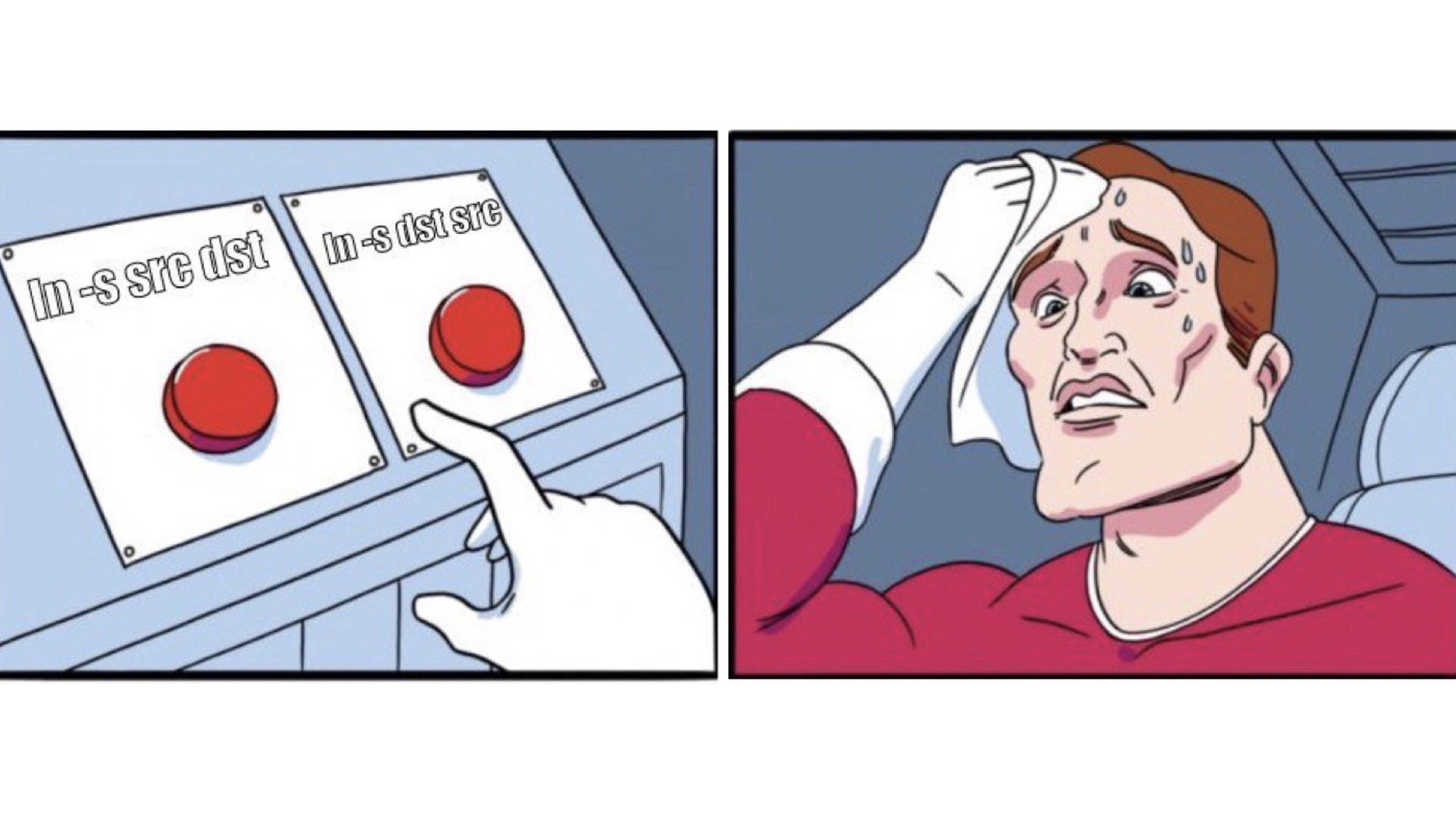
I hope you find inspirations from these pretty neat shell tricks and my shell setup.
Array Expansion
Use Array Expansion to quickly build arguments to a command. For example, to download several HTTP resources:
1
$ wget https://kubernetespodcast.com/episodes/KPfGep{001..062}.mp3
To rename files when you just want to change a substring.
1
$ mv /path/to/same-prefix-{old,new}.txt
Process Substitution and Anonymous Files
<()
redirects the stdout of the command in the subshell to a file descriptor opened at /dev/fd
. This is known as process substitution.
1
$ sh <(curl -sL https://istio.io/downloadIstioctl)
The above is equivalent to the following, where -
means to use the stdin as a file.
1
$ curl -sL https://istio.io/downloadIstioctl | sh -
Note that process substitution is more powerful than pipes, since you can do things like
1
$ diff <(ssh somehost cat /etc/hosts) <(ssh some-other-host cat /etc/hosts)
Start new shell
Just updated your bashrc/zshrc file, but want to hold on to your environment variables, file descriptors, etc? Do this.
1
$ exec -l $SHELL
The password is sudo
Dealing with production outage and in dire need of mitigation?
1
$ sudo su
And then you are always the root master. “I don’t test in prod; I dev in prod.”
Alias for the Win
Type less. Move fast. Here are some I use everyday.
1
2
3
4
5
6
7
8
mcd() { mkdir -p "$1" && cd "$1" }
cdl() { cd "$1" && ls }
alias ls='ls -F -G'
alias ll='ls -lh'
alias o='open'
alias ping='ping -c 5'
alias gauth='gcloud auth login --update-adc'
(I consider it a work injury that I now quote everything in shell and has long stopped using white space in file names even for personal stuff. Maybe you are like me.)
Cursor Navigation
Command Deletion
In-line file
1
2
3
4
5
6
7
8
9
10
cat << EOF | kubectl create -f -
apiVersion: v1
kind: Pod
metadata:
name: nginx
spec:
containers:
- name: nginx
image: nginx
EOF
Search Command History with Fuzzy Find
You probably know you could use ctrl+r
to retrieve a previously executed command quickly, but it only gives you one suggestion. fzf
solves it not only for commands but finds files as well.
Auto-correct Command Typos
I don’t endorse profanity but we all share the frustration. Thefuck will correct your mistake.
ZSH, ohmyzsh, and powerlevel10k
ZSH, or Z shell, is an extended version of the Bourne Shell (sh), with numerous new features, plugins, and themes. To change your shell to ZSH
1
$ chsh -s $(which zsh)
Ohmyzsh is a plugin manager for ZSH. I recommend at least two plugins.
autosuggestions
makes zsh like fish.
syntax-highlighting
colorizes the command and strings, providing visual warning for typos.